webpack + Moment.js
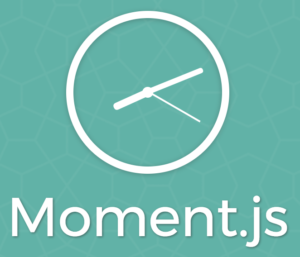
Moment.js is a powerful library that lets you “parse, validate, manipulate, and display dates and times in JavaScript”. Despite the recent emergence of other libraries like fecha and date-fns, Moment.js has reigned for a long time as the go to library for manipulating dates in JavaScript. Please do check out the features of these other lightweight alternatives if you have the opportunity. Unfortunately we are fairly entrenched with Moment because we’re working with a 3 year old code base. “It is, what it is”, so we just have to deal with it.
onto the problem
Our initial problem was that we couldn’t even get webpack to build moment into the bundle: from webpack-config/vendor.ts:
1 | import '../node_modules/moment/min/moment-with-locales'; |
grunt shell:webpackDev resulted in:
1 | WARNING in ./node_modules/moment/min/moment-with-locales.js |
The quick fix was to ignore the locale resolution, because moment-with-locales.js already had them:
1 | new webpack.IgnorePlugin(/\\.\\/locale$/, /moment\\/min/) |
But after looking at the BundleAnalyzer, we realized we were bringing in way too many locales. Much has been written about how to do this, so we stood on the shoulders of the giants and made the following changes: We tweaked vendor.ts to include moment instead of moment-with-locale and replaced the IgnorePlugin with this
1 | new webpack.ContextReplacementPlugin(/moment\[\\/\\\\]locale$/, /en|fr|es|zh|pt|nl|de|it/), |
and now our analyzer output looks like this:
140k to 29k !
And that’s the gzip comparison! SHIP IT! A quick update to our TODO list:
- Create vendor.min.js
- Create app-components.min.js
- Create app.min.js
- Create templates.app.min.js
- Try out webpack 3.0!
- Figure out what’s up with moment.js
- Use the webpack’d files in karma for unit tests
- Try the CommonChunksPlugin
- Handle CSS/LESS/SASS
- Use the dev-server instead of grunt-contrib-connect